Store
The Store
is the glue component that combines all other Suas components:
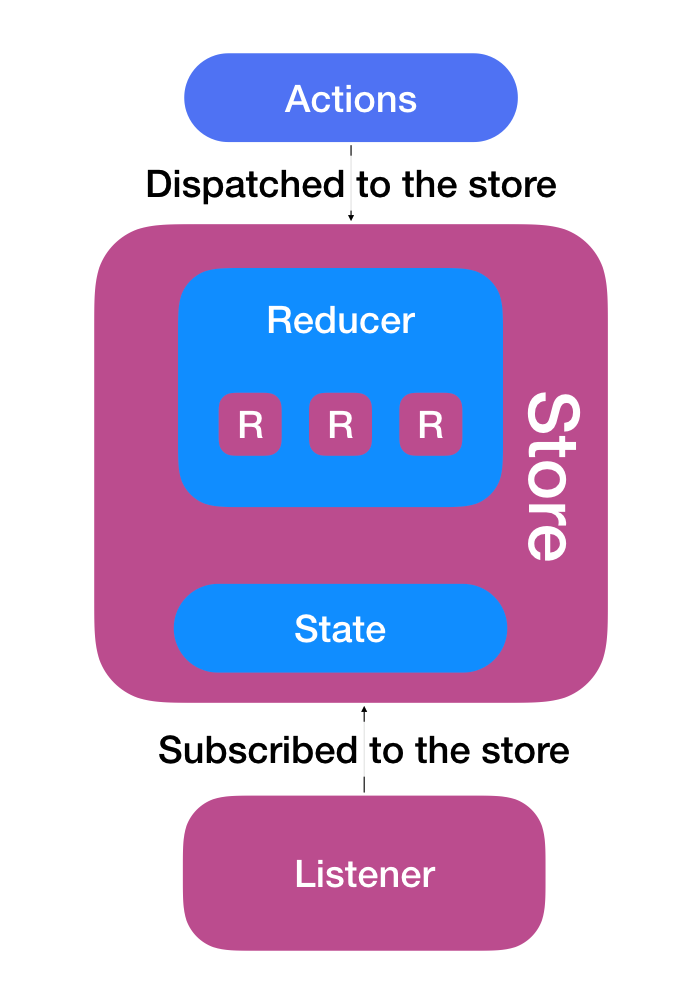
- Each application has one store only.
- The store contains the app state (or states)
- It also contains the app reducer (or combined reducers)
- Actions are dispatched to it. These actions will eventually cause state changes.
- Listeners are attached to the store. When state changes, these listeners are notified.
There are three steps to using the store:
- Creating a store.
- Dispatching actions.
- Listening for state changes.
Creating a store
We start by creating a store. The create store takes in the reducer (or group of reducers) and an optional list of middlewares.
In the todo app example we create a store with the TodoReducer
and the LoggerMiddleware :
let store = Suas.createStore(reducer: TodoReducer(),
middleware: LoggerMiddleware())
val store = Suas.createStore(TodoReducer())
.withMiddleware(LoggerMiddleware())
.build()
Store store = Suas.createStore(new TodoReducer())
.withMiddleware(new LoggerMiddleware())
.build();
Other parts of your application need to get a hold of this created store
in order to use it.
It is your responsibility to pass the
store
to your views. Some options are:
- Exposing
store
as a global singleton constant.- Using dependency injection to inject
store
in your views.
Note on more advanced usages
If your application contains multiple screens that are not logically or functionally tied; for example, in your todo app you might have a settings screen. In this case, instead of having a single reducer with two responsibilities we can instead combine to sub reducers and have a more modular reducer structure. Head to applications with multiple states to read more.
Dispatching actions
When some event occurs, such as tapping a button in a view or a network request has succeeded we create actions and dispatch them to the store. Dispatching Actions
is the only way to update the application state
that is held within the store
.
store.dispatch(action: AddTodo(text: "New Todo"))
store.dispatchAction(AddTodo(text = "New Todo"))
store.dispatchAction(getAddTodoAction("New Todo"));
The AddTodo
action is eventually sent to the reducer
by the store
.
Listening for state changes
Finally, to get updated when the state changes, we need to add listeners to the store.
// Adding a listener
store.addListener(forStateType: TodoSettings.self) { newState in
// Get called when the state changes
}
// Adding a listener
store.addListener(TodoList::class.java) { oldState, newState ->
// Get called when the state changes
}
// Adding a listener
store.addListener(TodoList.class, (oldState, newState) -> {
// Get called when the state changes
});
We will cover the listeners in more depth in the next section .
What's Next
Now that we created the store that joins all Suas components, it's time to add listeners to the store.
Related Topics
Also, check:
List of example applications built with Suas
Updated over 6 years ago